ASP.NET 2.0 Cross Page Posting
Introduction [Download Code]
In ASP.NET 1.X the default postback behavior is to postback the page to itself, but sometimes you need to post your page to another page in your application which was difficult in ASP.NET 1.x while it was so easy in ASP 3.0, so this missing feature is back to ASP.NET 2.0.
What The Problelm Is All About
I remember a problem that I have encountered before in ASP.NET 1.1, I was developing a checkout module in which the user is going through multiple pages to place an order, entering credit card, personal, and delivery information; the information was splitted on around 7 pages, and it was kinda wizard ( Thanks to Wizard Control in ASP.NET 2.0 ), so the user could move from one page to the other back and forth, but this information would be kept in one place and I have overriden the page submission through one java script snippet to post back to other page, that was simple, the challenge was how to expose the first page data to the recipient page, this actually could be done by more than one way, one is storing data into a cookie, other to store it into a user session on ther server, or to send them using Query strings parameters ( actually this is not a postback ! ), but by all the means the more pages you get the more complexity you will be in!
Handling Postbacks in ASP.NET 1.X
One of the commonly used properties in ASP.NET 1.X is Page.IsPostBack , it simply determines wheather it's a first request ( get ) , or a postback ( post ) operation, and it was really helpful for performance purposes, especially in cases of heavy data operations, for example if you have data grid that you populate when the page is loading and user selects one row, this selection required doing postback ( Thanks to AJAX ! ), so the page is flickering and also it's reloaded on the server, all the data are actually kept in the ViewState so there is no need to grab data again from database, here the importance of this magical property comes to light!
ASP.NET 2.0 Cross Page Postbacks
ASP.NET 2.0 came to make life of developers easier, and just to solve common problems that developers spend most of their time on, which consequently eating the project time. In ASP.NET 2.0 Cross Page Postbacks is so simple, actually more than simple, in the following lines you'll be shown almost everything you need to know about cross page postbacks in ASP.NET 2.0.
Practical Example
Run your VS.NET 2005, create a new web application, C# and it's preferred to leave defaults, remove the default page, and then add a page call it Personal Details.aspx, design the page as shown in the following picture
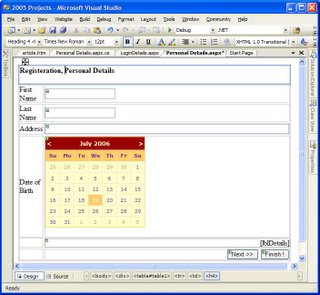
Rename the controls to whatever names you like, however you will find them named in a logical pattern, select the Next button, from properties window select property PostBackUrl and set it to LoginDetails.aspx , double click the Finish button to open the event handler method for click event of the button and enter the following code :
protected void btnFinish_Click(object sender, EventArgs e)
{
StringBuilder details = new StringBuilder();
details.Append("First Name : " + txtFirstName.Text + "<br/>");
details.Append("Last Name : " + txtLastName.Text + "<br/>");
details.Append("Address : " + txtAddress.Text + "<br/>" );
details.Append("Date of Birth : " + calDateOfBirth.SelectedDate.ToShortDateString());
lblDetails.Text = details.ToString();
}
As you can see above, this code is nothing new; you postback your page to itself, you read some control values then you concatenate everything then you display it in one lable.
Note : When you open your Login Details.aspx page code behind you will notice that your variables are not written to the code behind class as they were in ASP.NET 1.x, while they are just written while compiling your code, and this is the new Partial Classes approach introduced by ASP.NET 2.0, if you are not familiar with partial classes please refer to my blog post about Partial Classes.
Setting the PostBackUrl property doesn't imply you cannot add code for Click event handler, however still you can code to this button click event handler, after this code gets executed the page posts back to the second page, for demonstration purposes we've added the following code to event handler of the Next button, please double click the button and enter the following :
protected void btnSubmit_Click(object sender, EventArgs e)
{
Response.Write("<center><h2>Please Be Patient, You'll be Directed to Next Page Shortly !</h2></center>");
}
Now, we're done with the first page and it's the time to manage the recipient page, to process incoming post back data from the sender page, actually there is a new property of the Page Class called PreviousPage , and this property gets a reference to the page that gave control to the current page ( as described by MSDN ), and you can get access to all the members of a standard Page class, however we cannot write for example PreviousPage.txtFirstName.Text, this is basically because the controls are declared as Protected, so you've got 2 ways to do it, first is to use the mostly common used method FindControl , passing the control id and casting to the proper control data type, and second is to expose the controls that you need to use in second page as public properties, we'll introduce the second approach is it's more organized and object oriented, however the first one also works and it's a single line to be written.
Note : for the first approach you will need to write a line of code like this one,
TextBox postedFirstName = (TextBox)PreviousPage.FindControl("txtFirstName");
Then, you can use postedFirstName as a clone of the original txtFirstName at the first sender page.For the second approach we'll need to expose the controls as public read-only properties from the sender page, this one example for first name textbox :
public TextBox postedFirstName
{
}
You should do the same for all other controls you want to expose to your recipient page, however you will find the complete code in the downloadable sample attaching this article.
Now, go to the LoginDetails.aspx or the recipient page, and enter the following :
protected void Page_Load(object sender, EventArgs e)
{
lblDetails.Text = "First Name Entered in First Page is " + PreviousPage.postedFirstName.Text;
}
Try compiling your page; you will get a compile error described as : 'System.Web.UI.Page' does not contain a definition for 'postedFirstName', and this definately makes sense, as you're still in design time so how could the compiler know that postedFirstName is a member of the PerviousPage, so we have to supply something more to let the compiler aware of the PreviousPage data type, consequently you will be able to use the pretty bless of Intellisense.
Go the Source view of LoginDetails.aspx and add the following directive just after the main Page directive
This will tell the compiler and VS.NET Intellisense about your PreviousPageType, try compiling now the LoginDetails.aspx you will get no errors.
Note : Sometimes you use one page for more than one purpose, so in the above example LoginDetails page could be used as a standalone page away from the posting page, and at other times it could receive postback data from the first page, so you should distinguish between these 2 modes, to better handle events and postback data, you can edit the page load event handler in LoginDetails.aspx to be like this :
protected void Page_Load(object sender, EventArgs e)
{
if (Page.IsCrossPagePostBack)
{
lblDetails.Text = "First Name Entered in First Page is " + PreviousPage.postedFirstName.Text + "<br/>";
lblDetails.Text += "Last Name Entered in First Page is " + PreviousPage.postedLastName.Text + "<br/>";
lblDetails.Text += "Address Entered in First Page is " + PreviousPage.postedAddress.Text + "<br/>";
lblDetails.Text += "DOB Entered in First Page is " + PreviousPage.postedDateOfBirth.SelectedDate.ToShortDateString();
}
}
Now, you will only manage postback data if and only if the page is involved in a cross postback.
Warning : for some unknown reason you will always find the property Page.IsCrossPagePostBack false, I searched for the reason but I couldn't figure out why, also I found so many people wondering about this, unfortunatel I couldn't find and answer for this, it seems to be useless property, however you can work around this by reconstructing the condition to be like this :
if (Page.IsCrossPagePostBackPreviousPage != null)
As PreviousPage is not null in case of cross postback.
Conclusion
ASP.NET 2.0 Cross Posting is a great addition to ASP.NET 2.0, but the IsCrossPagePostBack property is somehow non-functional, while the PreviousPage property is always populated.
Labels: ASP.NET 2.0
<< Home